We recently run into a problem of having to display a vanilla JavaScript component in a React component. This component exposes a static method to render itself, like many of pure JS components do. In a “normal” JavaScript context it would be very easy to just call such a method, providing a DOM element to render itself into. However, when you want to render such a document as a part of your React component, this becomes a bit tricky. Read on to see how we solved this issue.
Table of Contents
Context
In our case, the component we wanted to render was CKEditor5. It’s a tiny and simple WYSIWYG editor.
Its documentation gives the following example on how to render the editor using a static create
function:
In our application, we have a React function component:
The goal is to render the ckeditor
component within the div
with id my-ckeditor
.
Can I call the component’s create function in React component?
The first idea that came to my mind was to somehow call the static create
function from ckeditor
providing my-ckeditor
as DOM element’s id. However, it turns out that we cannot do it directly in the function component before returning, because our div is not loaded into the DOM yet at this moment.
Fortunately, there’s another, easy solution ?
React on DOM element being mounted with useCallback
What we basically want to achieve is to execute the create
static function provided by ckeditor
when our div
is mounted into the DOM.
Let’s first install the ckeditor5 classic editor npm package:
npm i @ckeditor/ckeditor5-build-classic
Then require ckeditor.js
file as Ckeditor
in the .tsx file with our component:
const Ckeditor = require('@ckeditor/ckeditor5-build-classic/build/ckeditor.js');
Now, instead of assigning an id
to our div
element, let’s use the React.useCallback
hook and assign it to div
‘s ref
:
Our callback function will be called after our div
component mounts (or unmounts). That’s why we check whether the node
is defined, and then we can do something with that.
This is how it looks in debug mode:
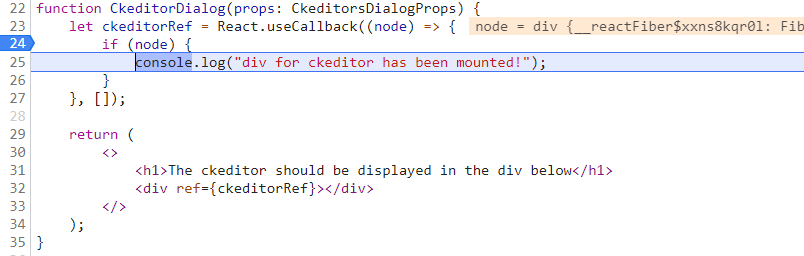
As you can see, node
is defined, and it’s a div
. Exactly the DOM element in which we want to render our ckeditor
component. Let’s now simply change console.log
to rendering the editor as per documentation:
Thanks to that, we can see our editor rendered on the page:
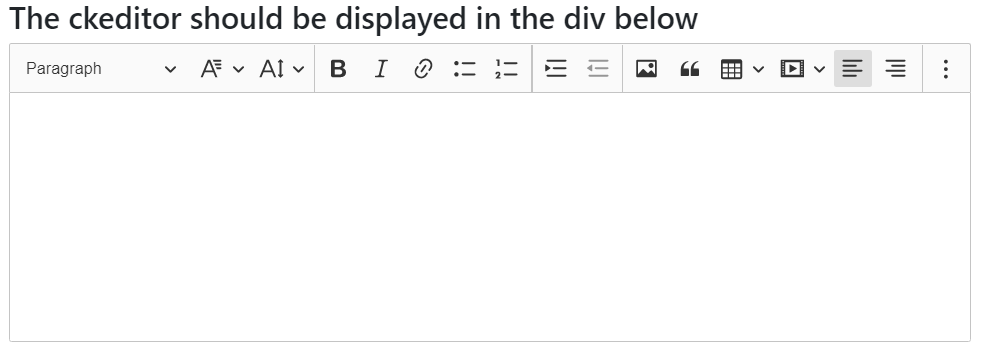
Summary
We often use external components in our projects at Yumasoft. In case of above-mentioned ckeditor
, we couldn’t use its React component (because of various reasons) and we had to render it statically using JS function. The solution with useCallback
turned out to be very useful here. I hope you also find it helpful one day if you ever need to use Javascript component in React ?