In one of our projects, we recently started using Cypress for end-to-end testing. Cypress executes tests written in JavaScript or TypeScript. However, we wanted to first perform some data-preparation operations using C#. In fact, we needed to run Cypress tests from NUnit C# tests.
In this article, I’m sharing how this can be done ? It will also be useful if you use another testing framework than NUnit, but the examples are based on it.
Table of Contents
Starting point
You can find the code accompanying this article here: https://github.com/dsibinski/cypress-csharp-example
Our starting point is a React application with Cypress tests:
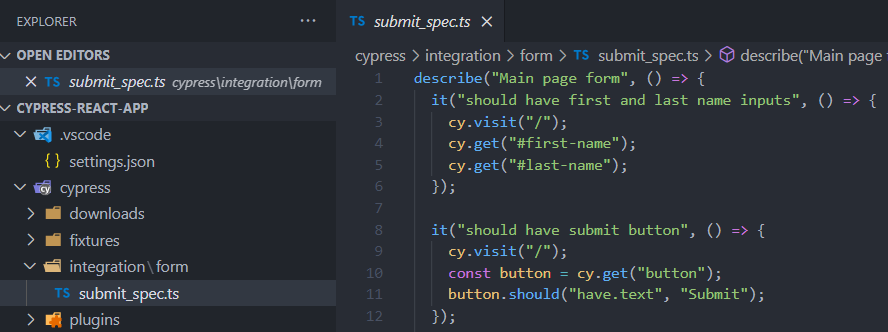
I assume that your tests specs are placed within cypress/integration
folder. The only spec we have is called submit_spec.ts
and it placed in a subfolder called form
.
Now imagine, that your backend is written in C#. Maybe you’d like to perform some data initialization before Cypress tests are executed.
In our case, we have a C# backend, and we’ve already had a mechanism for running integration tests. This mechanism runs our server app with an in-memory database, which makes the maintenance of tests very easy.
We wanted to use the same mechanism for our new, E2E Cypress tests. It means that we would be able to write some C# initialization code (data creation mostly) and then run Cypress tests from NUnit test.
What we want to achieve is to have a C#-Cypress NUnit test look as follows:
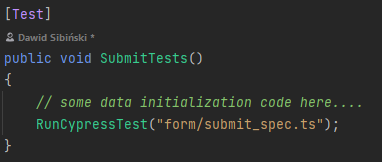
Let’s now see how we can implement the RunCypressTest
method ?
Implementing a method to run Cypress tests from NUnit
To make things easier, let’s create a base class all our test classes can use. We’ll call it CypressTestsBase
and add a RunCypressTest
method to it:
This code does the job. Few interesting parts:
- Line 12 – I’m getting there the path to the web app workspace where
npx cypress run
command can be executed. It might be more complicated in your scenario, so you should adjust accordingly here - Lines 13-20: thanks to setting
RedirectStandardInput
totrue
, we can later execute a custom command viacmd
; settingRedirectStandardOutput
totrue
allows us to write everythingnpx cypress run
command does to NUnit test’s output - Line 28:
TestContext.Out.WriteLine(cypressProcessOutput);
actually writes the Cypress output to NUnit test result - Line 29: here we handle errors during Cypress execution.
npx cypress run
executed viacmd
will return a code different from 0 if anything goes wrong.
We can now change FormTests
class to run its corresponding Cypress test:
Finally, it allows to run our C# test, which then runs its corresponding Cypress spec and prints the output:
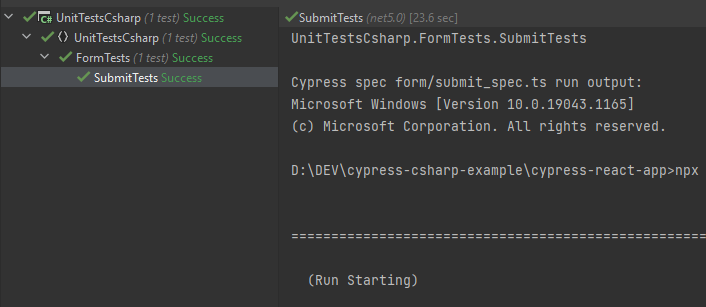
Cypress errors in NUnit result
Let’s see what happens if something is broken on Cypress/web app’s side. Let’s run our test, but with our web app not running.
The NUnit test fails, and we can clearly see what was wrong:
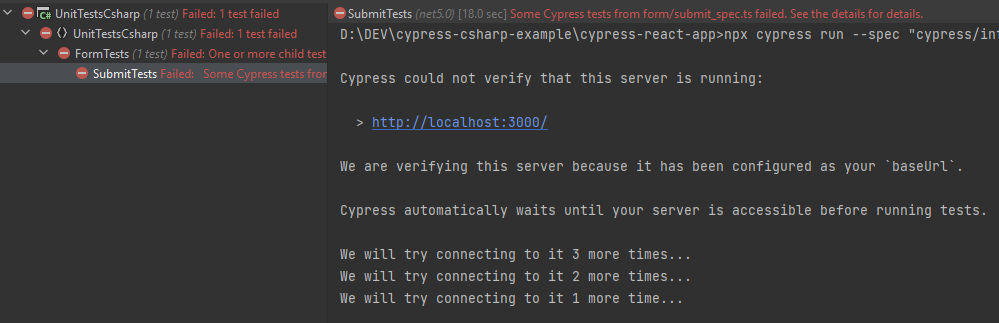
You will see similar errors if something goes wrong in a Cypress test. Cypress also saves compressed videos and screenshots, so if something goes wrong, diagnosing such failed tests is very easy.
Summary
I hope you found this article on how to run Cypress tests from NUnit useful. Remember that it not only works with NUnit – it will be suitable for any testing framework. You might need to adjust some parts of the solution, e.g. printing the results to the test result’s output.
This solution allowed us to improve our testing by adding a new type of tests to our pipeline. Right next to proper naming and mutation testing, E2E tests add another layer of security and improve the quality of our software.
Once more, you can find the complete source code here. If you want, feel free to copy it and change it according to your needs ?
Great article
thanks!
process.StandardOutput.ReadToEnd();
the output I am getting using this is in some strange language.
ΓöîΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÉ
Γöé Cypress: 12.7.0 Γöé
Γöé Browser: Electron 106 (headless) Γöé
Γöé Node Version: v16.17.0 (C:\Program Files\nodejs\node.exe) Γöé
Γöé Specs: 1 found (spec.cy.js) Γöé
Γöé Searched: C:\Users\Admin\Documents\NewApproach_3LAyer\cypress\e2e\spec.cy.js Γöé
ΓööΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÿ
ΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇΓöÇ
what can be done to solve it.